【Android】Jetpack Compose 入門講座 第14回「リストのスクロール①」
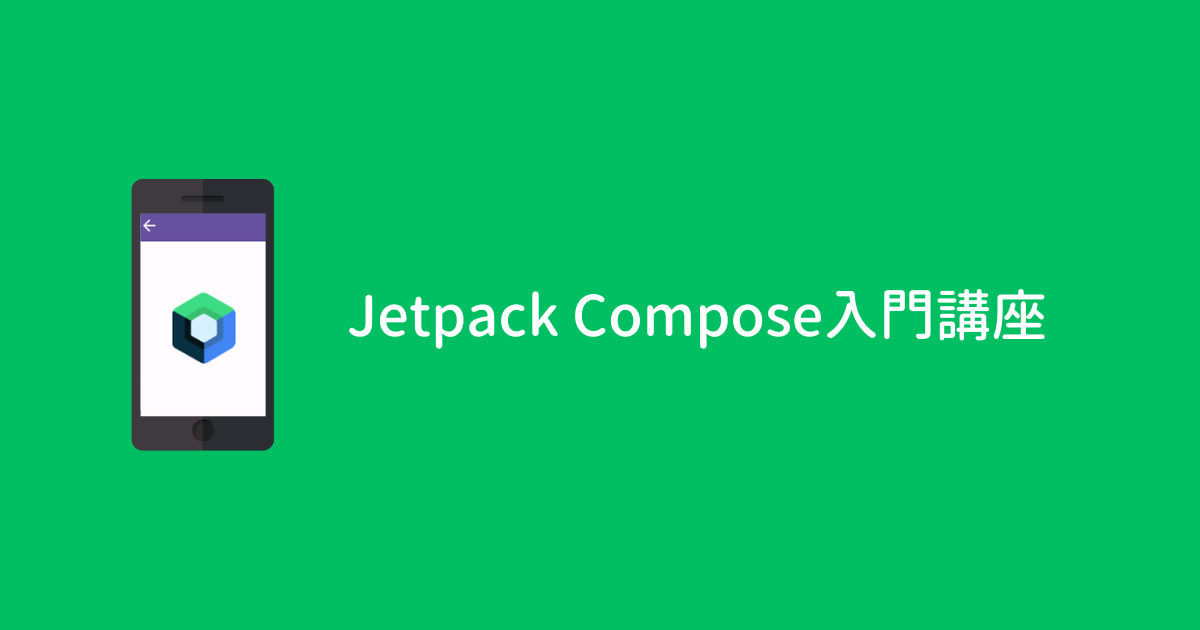
はじめに
今回は動物のリストをスクロールできるようにします。
またエミュレータでアプリを実行しなくても動作を確認できる Interactive Mode(インタラクティブモード)も紹介します。
スクロール設定
コードの追加
動物リストをスクロールできるようにするには、たった1行コードを追加するだけです。
AnimalListRow 関数の Row 部分に4行目のコードを追加します。
@Composable
fun AnimalListRow(modifier: Modifier = Modifier) {
Row(
modifier = modifier.horizontalScroll(rememberScrollState())
) {
AnimalListElement(drawable = R.drawable.dog, text = "Dog")
今回は水平方向(Horizontal)のスクロールなので horizontalScroll と書きましたが、垂直方向のスクロールには verticalScroll を使用します。
プレビュー関数の追加
AnimalListRow 関数用のプレビューも用意しておきましょう。
7〜11行目を追加して、13行目に書いていた widthDp = 480 は削除します。
@Preview
@Composable
private fun AnimalListElementPreview() {
AnimalListElement(drawable = R.drawable.dog, text = "Dog")
}
@Preview
@Composable
private fun AnimalListRowPreview() {
AnimalListRow()
}
@Preview
@Composable
private fun MyAppPreview() {
MyApp()
}
Interactive Modeで確認
プレビュー画面にある Interactive Mode(インタラクティブモード)という機能を使うと、エミュレータを起動しなくてもスクロールやタップの動きを確認することができます。
プレビュー画面をクリックして、右上に表示される Start Interactive Mode をクリックします。
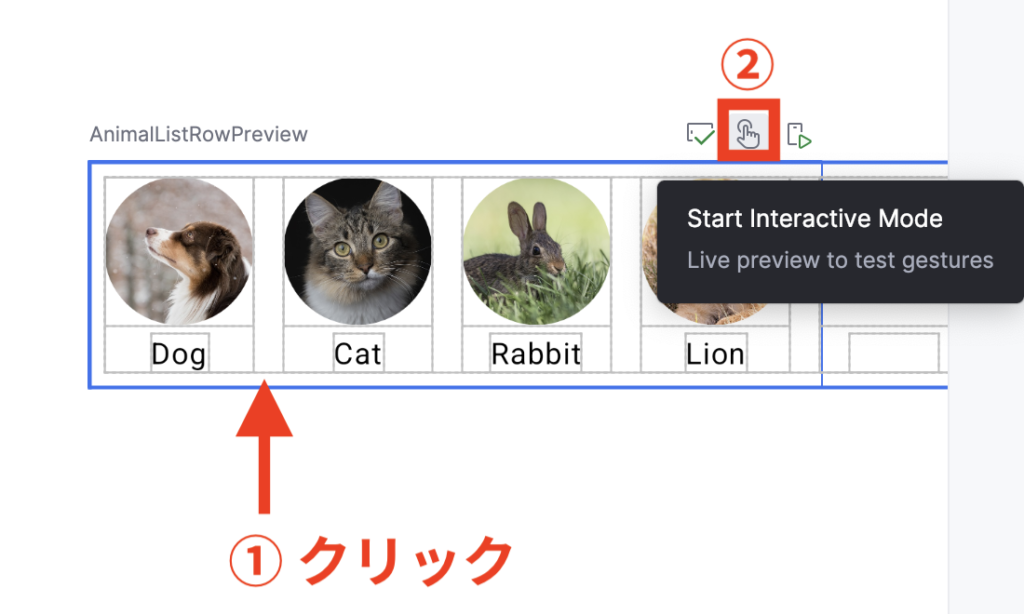
プレビュー上でスクロールできるようになります。
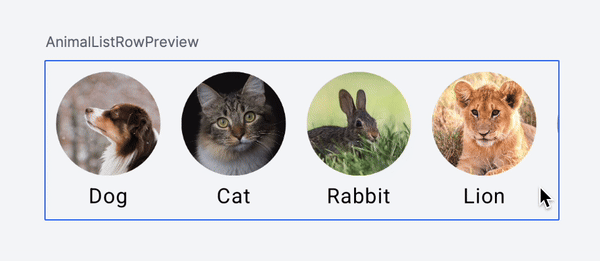
確認が終わったらプレビュー画面左上にある Stop Interactive Mode をクリックして停止します。
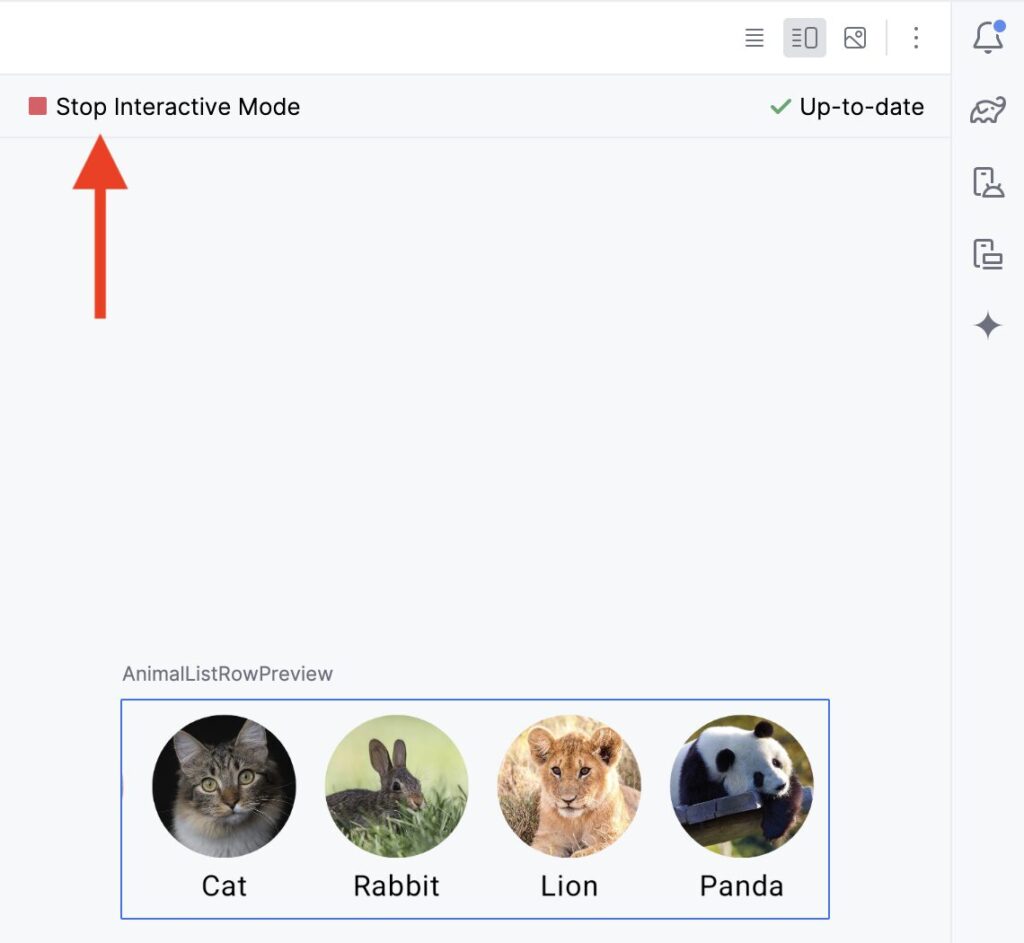
次に行うこと
今回は表示するデータ数が少ないので良いですが、データ数が多くなるとデータを一度に読み込むと時間がかかってしまいます。
次回は効率的にデータを表示する方法を紹介します。
ここまでのコード
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.activity.enableEdgeToEdge
import androidx.annotation.DrawableRes
import androidx.compose.foundation.Image
import androidx.compose.foundation.horizontalScroll
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.size
import androidx.compose.foundation.layout.statusBarsPadding
import androidx.compose.foundation.rememberScrollState
import androidx.compose.foundation.shape.CircleShape
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
enableEdgeToEdge()
setContent {
MyApp()
}
}
}
@Composable
fun MyApp(modifier: Modifier = Modifier) {
MyApplicationTheme {
Surface(
modifier = modifier
.fillMaxSize()
.statusBarsPadding()
) {
AnimalListRow()
}
}
}
@Composable
fun AnimalListElement(
@DrawableRes drawable: Int,
text: String,
modifier: Modifier = Modifier
) {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = modifier.padding(8.dp)
) {
Image(
painter = painterResource(id = drawable),
contentDescription = text,
contentScale = ContentScale.Crop,
modifier = modifier
.size(80.dp)
.clip(CircleShape)
)
Text(
text = text,
modifier = modifier.padding(top = 4.dp)
)
}
}
@Composable
fun AnimalListRow(modifier: Modifier = Modifier) {
Row(
modifier = modifier.horizontalScroll(rememberScrollState())
) {
AnimalListElement(drawable = R.drawable.dog, text = "Dog")
AnimalListElement(drawable = R.drawable.cat, text = "Cat")
AnimalListElement(drawable = R.drawable.rabbit, text = "Rabbit")
AnimalListElement(drawable = R.drawable.lion, text = "Lion")
AnimalListElement(drawable = R.drawable.panda, text = "Panda")
}
}
@Preview
@Composable
private fun AnimalListElementPreview() {
AnimalListElement(drawable = R.drawable.dog, text = "Dog")
}
@Preview
@Composable
private fun AnimalListRowPreview() {
AnimalListRow()
}
@Preview
@Composable
private fun MyAppPreview() {
MyApp()
}